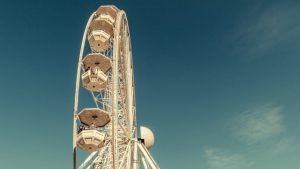
I think one of many reasons it’s so hard to learn to code is that the people doing the teaching have all forgotten what it was like not to know how to code, so we skip over some really important basic steps because we think they’re inherently obvious. Case in point, this poor redditor who nobody ever taught how to work through a piece of code with a pencil and paper. I had to read the question a couple of times to understand what they were even asking, it just didn’t occur to me that someone wouldn’t know how to trace through code manually.
The example that user posted was “x=0; y=0 While (x<7) { x=y-x; y=x+x; x= x+ y + 1;}”
First of all, let’s clean that up a little:
x=0; y=0; while (x < 7) { x = y - x; y = x + x; x = x + y + 1; }
In the beginning x is 0, which is definitely less than 7 so we enter the loop.
Inside the loop, take each statement and swap out the variables for their current values and then do the math and put that value in the variable:
x = y – x becomes x = 0 – 0 becomes x = 0
y = x + x becomes y = 0 + 0 becomes y = 0
x = x + y + 1 becomes x = 0 + 0 + 1 becomes x = 1
At the end of the loop x = 1 and y = 0
Now we check the condition at the top of the loop again and x is still less than 7 so we go through the loop again
x = y – x becomes x = 0 – 1 becomes x = -1
y = x + x becomes y = -1 + -1 becomes y = -2
x = x + y + 1 becomes x = -1 + -2 + 1 becomes x = -2
At the end of the loop x = -2 and y = -2
At this point, I’m getting very suspicious this loop will never exit. I don’t think x is ever going to be greater than or equal to 7, but just to be sure let’s go through the code one more time
x is still less than 7 so we go through the loop again
x = y – x becomes x = -2 – -2 becomes x = 0
y = x + x becomes y = 0 + 0 becomes y = 0
x = x + y + 1 becomes x = 0 + 0 + 1 becomes x = 1
At the end of the loop x = 1 and y = 0. These are the same values we had after we went through the loop the first time, so now we can be completely sure the loop never ends. After each time through the loop x is either 1 or -2, there’s no way for it ever to be 7 or more.
That’s it. Working through code with a pencil and paper is just keeping track of what values all of your variables have and swapping out variables for their values. It’s really tedious, that’s why we make computers do it :) And to be clear, you’re never expected to be able to work through complicated code like that purely in your head. Everybody else needs a pencil and paper too, there are just too many details to keep them all in your working memory.
Okay, so if working through code with a pencil and paper sucks so much, why does anyone do it? Because it gives you a really solid understanding of what the computer is doing, which you’ll need later when you start building more complicated things. For a simple loop like the one above you can just throw that snippet of code into an IDE, run it, and see what happens, but you can’t even start building bigger things without a foundation of knowing what the computer is doing when it runs your code.
1 Comment
What does reasoning about code even mean? – Mel Reams
[…] Programmers talk about “reasoning about code” all the time, but what does that actually mean? Is it really just pretending to be a CPU and working out exactly when your for loop ends? […]