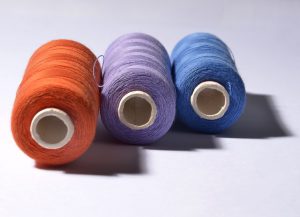
Threads are used a lot in java, so I should probably understand how they actually work. They’re one of those things you use all the time without thinking about what’s really happening under the covers. I know threads and processes are related, but not exactly how.
First of all, what’s a process? To understand threads inside a process you need to understand processes first.
A process is an individual thing that’s separate from all the other processes running on your computer. In most cases a process is a single program running on your computer, but some programs have many processes – Chrome, for example, has a process for each tab. To quote the official tutorial on concurrency in Java: “A process has a self-contained execution environment. A process generally has a complete, private set of basic run-time resources; in particular, each process has its own memory space.” As I understand it, the separate memory space is the really important part of a process – this keeps processes from overwriting each other’s memory, crashing your computer, and wrecking your day.
Threads, on the other hand, are just parts of the parent process that execute semi-separately and all use the same memory. This means they can change a variable another thread just set, cause wildly bizarre bugs, and wreck your day. Every process has at least one thread, but can have more.
Okay, so why would you want to use threads when you could use processes to keep everything separate? Because processes use up a lot more system resources than threads, it’s more work to get them to communicate with each other, and they’re just overkill for a lot of problems. If you’re building in a search feature in your app and you want to search by a few different things (like name, address, and ID number) from one search box, creating a whole process for each search is way more work than you need to do when you could just use threads. Do you really want to write a whole separate program for each search? I sure don’t.
So threads are convenient, but what are they really? Saying they’re a single thread of execution through a program is all well and good, but what does that really mean? To quote stackoverflow: “A thread is a basic unit of CPU utilization; it comprises a thread ID, a program counter, a register set, and a stack.” Those things are also called the execution context, because they’re everything you need to know about the executing program to stop it and start it again in exactly the same state it was in. The CPU does that a lot so it can appear to do two things at once (if you have a multicore processor, it actually can do more than one thing at once). If one thread is waiting for data to come back from the database, which takes forever from the perspective of a CPU, the CPU will essentially make a note of where the thread got to in the code and what values all of its variables had, then start executing another thread using the “notes” it made about that one.
Because threads all share the same address space, they can share information really easily by updating global variables. In a process, a memory address (aka a variable) will probably mean nothing to another process. In a thread, any variable that’s in scope can be used and (if it’s not final) changed. This is really handy for stuff like web programming – if a thread that’s handling a particular HTTP request needs a reference to the database service, it can just grab one from the controller/servlet/general parent object that contains methods for handling individual requests.
When a thread completes, its variables get garbage collected as usual (assuming nothing else has a reference to them), you don’t have to do anything special to clean up after it.
And finally, while the tutorial I linked above talks about Thread objects and Runnables, in modern Java you mostly use CompletableFutures and let them handle starting and stopping threads for you.