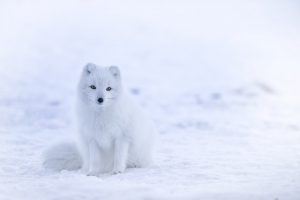
Last time I mentioned that there are bounded generic types and wildcards. I’ve never actually used one of those if I remember correctly, which I why I left them for a followup post.
Bounded generics are really cool – they let you narrow down which types you can use with a generic class or method while still having the flexibility to work with different types in the range you’ve defined. There are lower bounded and upper bounded generics. Upper bounded generics specify a superclass your generic type must extend and lower bounded generics specify a class your generic class must be a superclass of.
This means that you can do more interesting stuff with your generic param because you know something about what it is. Let’s use WordPress as an example. If you had a theme superclass (or probably an interface) that was extended or implemented by, say, oneColumnTheme, twoColumnTheme, gridLayout, etc you could have a theme preview page that accepts anything that extends the base theme class and calls getPreviewImage and getDescription on every item passed in, even if they’re different subclasses.
That method signature would look like:
public Result createPreviewPage(List<? extends Theme> themeList) { //code goes here }
You can’t add anything except null to a collection that has an upper bounded generic type, though. Why? Java inheritance – if you have a class hierarchy of Vehicle -> Car -> Sedan -> Toyota Corolla then that Toyota is definitely a Sedan, a Car, and a Cehicle, but a Vehicle could very well be a Motorcycle or a Bus, not any variety of Car.
On the lower bounded end of bounded generics, you could have a method that takes anything that’s a superclass of integer (which could be a number or object) and adds all the numbers from 1 to 10 to it. Yes, I stole this example (and the following code) from the official docs. The code for that method looks like:
public static void addNumbers(List<? super Integer> list) { for (int i = 1; i <= 10; i++) { list.add(i); } }
With a collection with a lower bounded type you can add things. Taking my Vehicle -> Toyota Corolla class hierarchy above, if you have a List<? super ToyotaCorolla> then you know what the most specific thing it can be is so you can safely add one of those.
You have have a lower bound or an upper bound but not both. You can have neither, though! That’s called an unbounded wildcard and it looks like:
public boolean removeAll(Collection<?> c);
That’s from the List source code. Unbounded wildcards are for when you really just don’t care what the thing is and when you want to make sure you don’t make any major changes to your parameter. The only thing you can do with a Collection<?> is add null, you just don’t know enough about what’s in there to add an object or any sort.
In my admittedly limited experience with bounded generics, upper bounded generics are much more common so you shouldn’t be too worried if you can’t find a use for a lower bounded generic parameter. If you’re not sure when to use which kind of wildcard/bounded generic, there’s a handy guide in the official docs. There are also some really in-depth articles about generics here and here if you’d like much, much more detail :)