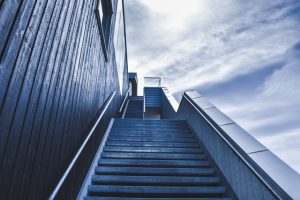
As part of my ongoing effort to demystify development, let’s talk about what personality traits actually matter even a little bit for developers.
There are tons of stereotypes out there about how you have to be super smart or great at math or have started programming when you were six to be a really good programmer. That’s all total bullshit.
I believe there is a single personality trait that’s extremely important to becoming a programmer, but it has nothing to do with how smart you are.
Intelligence, in as much as you can even measure something so poorly defined, simply does not matter. In my experience being clever has nothing to do with whether your code is readable or maintainable. I would much rather work with someone who knows they aren’t a genius and codes to avoid common mistakes than someone who is in love with how smart they think they are.
Being great at math can be helpful, but it’s by no means necessary. Math is really good practice at thinking through a problem one step at a time, particularly stuff like proofs, but if you’re not working on games or graphics programming you may never use any math more complicated than basic arithmetic in your day to day job.
Having started programming when you were very young can also be helpful because that gives you more years of experience, but it’s not as if there aren’t plenty of great programmers who never touched a computer until high school or even college.
So if none of those things really matter, what does? Stubbornness. I firmly believe the one personality trait you really need to be a programmer is stubbornness.
Programming, particularly when you’re just starting out, can be immensely frustrating. Teeny tiny little details like where exactly you put that semi-colon will ruin your day. The sheer amount of stuff you need to learn will seem totally overwhelming. You will feel stupid and think about quitting over and over and over.
Learning to code these days with friendly modern languages like python is certainly easier than learning assembler back in the day, but you need to be pretty stubborn to learn enough programming to be able to build something interesting.
But don’t think it’s all smooth sailing once you learn to code. Just the other day I spent a couple of hours debugging what I thought was an issue in my server code, but was actually me passing in the wrong parameter names through a javascript api like a dope. The more time you spend programming, the more horrible bugs you will run into, the more tough design problems you will have to solve, the more times you’ll have to figure out how to refactor existing code to get something new working, the more times you will kick yourself when the thing that seemed like a great idea six months ago turns out to be a terrible idea after all, and so on and so on.
As much as I love programming, I still think that you have to be a little bit weird to put up with this level of frustration. If programmers had the sense to do something that was less of a pain in the ass, they wouldn’t be programmers :)